[참고]
- 옥수별 [33편] 허프변환을 이용한 원 찾기 : https://blog.naver.com/PostView.nhn?blogId=samsjang&logNo=220592858479&proxyReferer=https%3A%2F%2Fwww.google.com%2F
- Hough Circle Transform (gramman readthedocs) : https://opencv-python.readthedocs.io/en/latest/doc/26.imageHoughCircleTransform/imageHoughCircleTransform.html
- 파이썬과 openCV를 이용한 컴퓨터 비전학습 / 알렉세이 스피쉐보이, 알렉산드르 류브니코프 저 / T4 역 / 에이콘 출판
- 멈춤보단 천천히라도 (블로그): https://webnautes.tistory.com/949
1. 원, 직선 그리기
[코드]
import cv2
import numpy as np
img = np.zeros((500,500), np.uint8)
cv2.circle(img, (200,200), 50, 255, 3)
cv2.line(img, (100, 400), (400, 350), 255, 3)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
[결과]
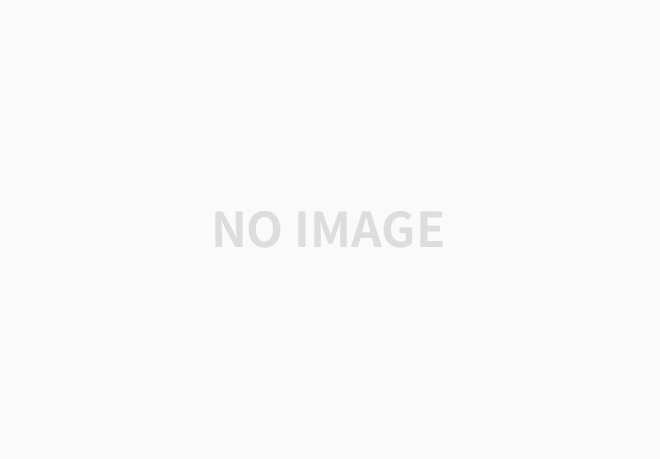
2. 직선 좌표 구하기
import cv2
import numpy as np
img = np.zeros((500,500), np.uint8)
cv2.line(img, (100, 400), (400, 350), 255, 3)
lines = cv2.HoughLinesP(img, 1, np.pi/180, 100, 100, 10)[0]
dbg_img = np.zeros((img.shape[0], img.shape[1], 3), np.uint8)
for x1, y1, x2, y2 in lines:
print('Detected line: ({} {}) ({} {})'.format(x1,y1,x2,y2))
cv2.line(dbg_img, (x1,y1), (x2,y2), (0,255,0),2)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
[결과]
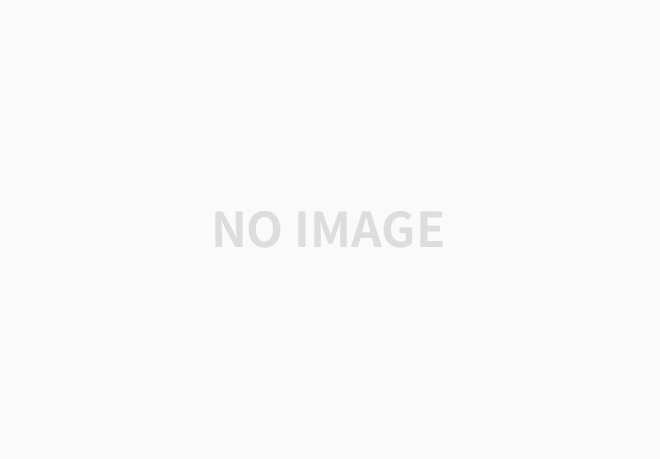
Detected line: (100 402) (401 349)
3. 원 중심, 반지름(raidus) 구하기
[코드]
import cv2
import numpy as np
img = np.zeros((500,500), np.uint8)
cv2.circle(img, (200,200), 50, 255, 3)
circles = cv2.HoughCircles(img, cv2.HOUGH_GRADIENT, 1, 15, param1 = 200, param2 = 30)[0]
dbg_img = np.zeros((img.shape[0], img.shape[1], 3), np.uint8)
for c in circles:
print('Detected circle: center = ({} {}), radius = {}'.format(c[0],c[1],c[2]))
cv2.circle(dbg_img, (c[0], c[1]), c[2], (0,255,0), 2)
cv2.imshow('img', img)
cv2.waitKey()
cv2.destroyAllWindows()
[결과]
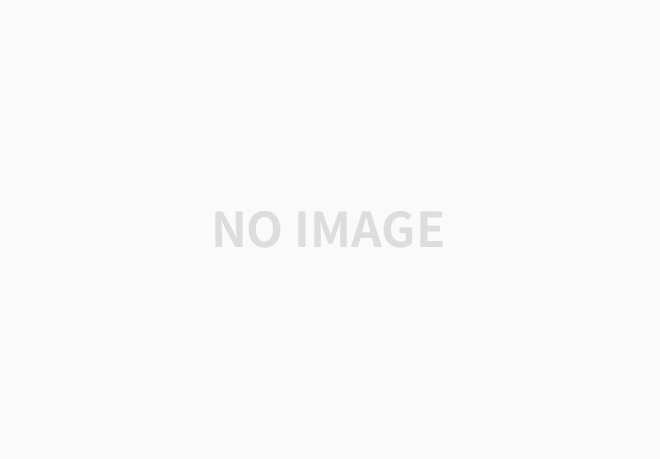
Detected circle: center = (199.5 199.5), radius = 47.29999923706055
4. 이미지로부터 원의 중심점 찾기
[코드]
import cv2
import numpy as np
img = cv2.imread('do.jpg')
img = cv2.medianBlur(img, 5)
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
circles = cv2.HoughCircles(img, cv2.HOUGH_GRADIENT, 1, 15, param1 = 200, param2 = 30)[0]
dbg_img = np.zeros((img.shape[0], img.shape[1], 3), np.uint8)
for c in circles:
print('Detected circle: center = ({} {}), radius = {}'.format(c[0],c[1],c[2]))
cv2.circle(dbg_img, (c[0], c[1]), c[2], (0,255,0), 2)
cv2.imshow('img', img)
cv2.imshow('dbg_img', dbg_img)
cv2.waitKey()
cv2.destroyAllWindows()
[결과]
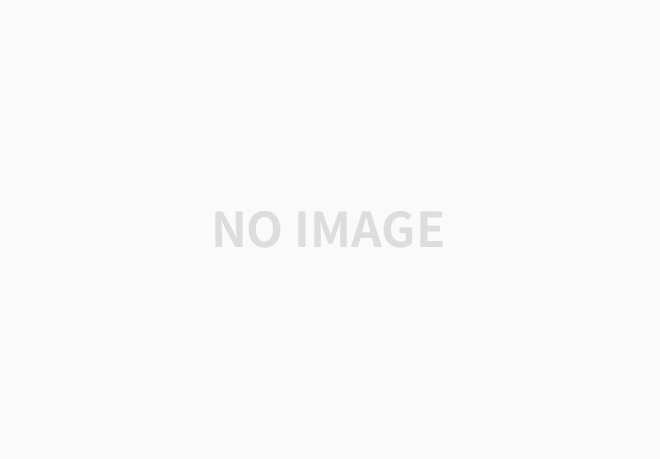
Detected circle: center = (319.5 319.5), radius = 57.400001525878906
Detected circle: center = (212.5 55.5), radius = 49.599998474121094
'#openCV # 파이썬' 카테고리의 다른 글
#dlib 설치 #python3.6 (0) | 2019.05.02 |
---|---|
#openCV #python #카메라 autofocus 기능 끄기 (0) | 2019.04.29 |
#openCV #영상분할 #cv2.threshold(), cv2.inRange() (0) | 2019.04.04 |
#openCV #이미지 프로세싱 #cv2.cvtColor(), cv2.inRange() (0) | 2019.04.04 |
#openCV #Image Contours #cv2.findContours() #cv2.drawContours() (0) | 2019.04.03 |